Table of contents
Prisma.js is a modern Object-Relational Mapping (ORM) tool that allows developers to manage databases easily and efficiently. Prisma.js allows you to create and modify database schemas in TypeScript or JavaScript.
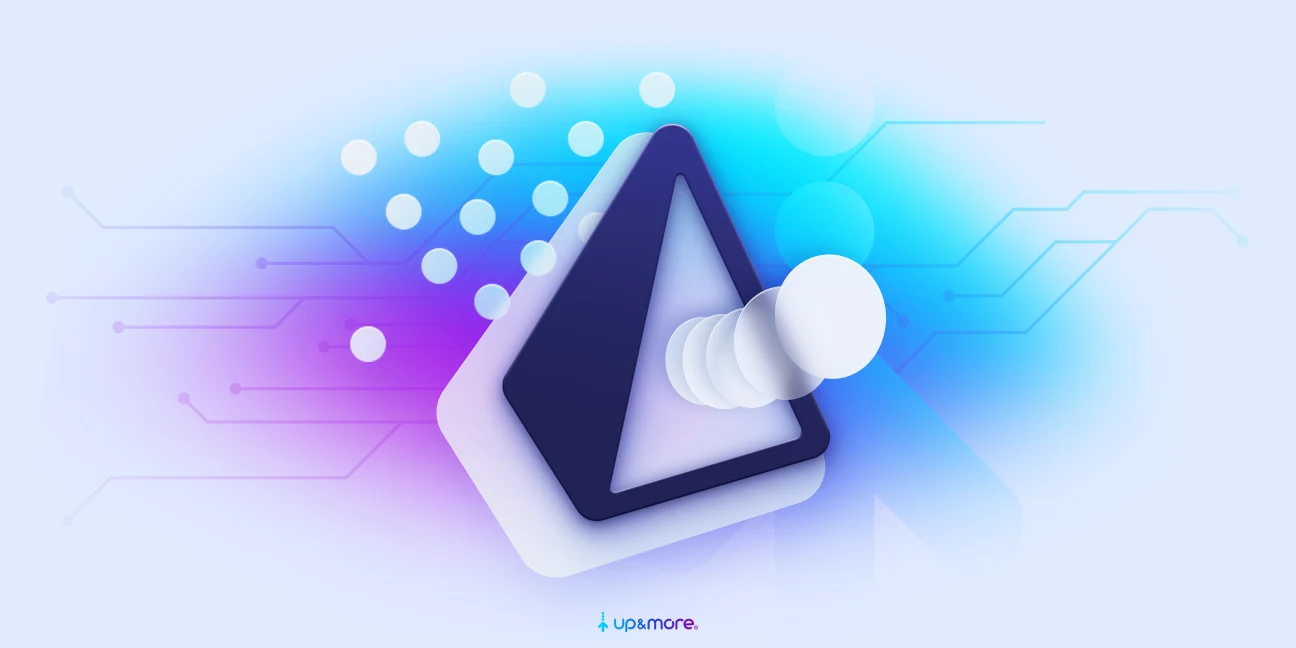
Prisma.js offers many advantages, including:
- Data security: Prisma.js ensures data security by using strong typing and validation of input and output data.
- Efficiency: Prisma.js uses modern technologies and fast algorithms to ensure fast and efficient database operations.
- Elegant code: Prisma.js enables you to write elegant and readable code by using a declarative API.
The basics of Prisma.js involve defining a database schema in TypeScript or JavaScript, and then generating source code for the database models. Prisma.js makes it easy to create, update and delete data in the database using an elegant API.
Prisma.js is a tool that is fully compliant with the modern requirements of full-stack projects, allowing developers to fully focus on application development rather than worrying about database management. Prisma.js works with many popular databases, including PostgreSQL, MySQL and MongoDB.
Prisma.js also offers a lot of advanced functionality, such as handling relationships between tables, transactions and event handling. In addition, Prisma.js allows you to easily scale your application by taking advantage of database clustering.
If you are a developer looking for a modern and effective ORM tool, then Prisma.js is an ideal choice. With this tool, you will be able to fully focus on developing your application, and not worry about database management.
It will allow you to develop your application with ease.
Create data models with Prisma.js
Prisma.js allows you to easily define data models using TypeScript or JavaScript. To define a data model, simply create a prisma file that contains the model definition.
For example, to create a user model, you can create a User.prisma file with the following content:
model User {
id Int @id @default(autoincrement())
name String
email String @unique
posts Post[]
}
model Post {
id Int @id @default(autoincrement())
title String
content String?
author User? @relation(fields: [authorId], references: [id])
authorId Int?
}
In the above example, the User and Post models are defined. The User model contains the fields id, name, email and posts The id field is set as a primary key using the @id annotation, and as an autoincrement field using the @default(autoincrement()) annotation. The email field is set to be unique using the @unique annotation. The User model also has a relationship with the Post model via the posts field.
The Post model contains the fields id, title, content, author and authorId The id field is set as a primary key and auto-increment field, similar to the User model. The author field establishes a relationship with the User model via the @relation annotation. The authorId field is a foreign key that references the id field of the User model.
After defining the data models, you can generate the source code for the models using the Prisma CLI tool. Simply use the prisma generate command to generate the source code for the data models.
Provided that you can use the prisma generate command to generate the source code for the data models.
npx prisma generate
With this Prisma.js tool, you can easily manage your database with a clear and elegant API.
Working with databases – queries and relationships in Prisma.js
Prisma.js allows you to easily create database queries using a clear and intuitive API. For example, to retrieve all users from the database, simply call the findMany function for the User model:
const users = await prisma.user.findMany();
If we want to retrieve a specific user based on their ID, we can use the findUnique function:
const user = await prisma.user.findUnique({
where: {
id: 1
}
});
Prisma.js also allows you to easily create queries using conditions, sorting and pagination. For example, to retrieve users from the database who have an email address containing the word “gmail.com“, we can use the findMany function using filter:
const users = await prisma.user.findMany({
where: {
email: {
contains: "gmail.com"
}
}
});
Prisma.js also provides support for relationships between tables. To retrieve all posts written by a given user, we can use the findMany function using filtering:
const user = await prisma.user.findUnique({
where: {
id: 1
},
include: {
posts: true
}
});
In the above example, we retrieve a user with ID 1 along with his posts. By using the include parameter in the findUnique function, we can retrieve related records from another model.
Prisma.js also makes it easy to create relationships between records in the database. For example, to add a new post to the database and assign it to a particular user, we can use the create function:
const post = await prisma.post.create({
data: {
title: 'New post',
content: "Lorem ipsum dolor sit amet...",
author: {
connect: {
id: 1
}
}
}
});
In the above example, we create a new post and assign it to a user with ID 1 using the connect parameter. With this Prisma.js tool, you can easily create and manage relationships between records in the database.
Migrations in Prisma.js – managing changes in database schema
Migrations in Prisma.js allow you to easily manage changes to the database schema. To create a migration, use the prisma migrate save command, which will create a migration file containing changes to the database schema. Then, to apply the migration to the database, use the prisma migrate up command. Prisma.js also allows you to undo the migration using the prisma migrate down command.
With this tool, you can easily update the database schema and maintain data integrity in the database. Prisma.js also offers many advanced features, such as support for database schema versioning and automatic generation of migrations based on changes in data models.
Was the article helpful?
Rate our article, it means a lot to us!
Let's talk!
I have been passionate about technology and programming for many years. I specialize in JavaScript and TypeScript, which allows me to create dynamic and flexible web applications. Over the course of my career, I have gained experience in a variety of projects, from simple websites to complex systems integrated with databases. I'm also a fan of JAMStack technology, which allows me to create fast, secure and scalable applications, which is crucial for me when it comes to the quality and usability of the products I create. In my free time, I love experimenting with new technologies and constantly evolving.